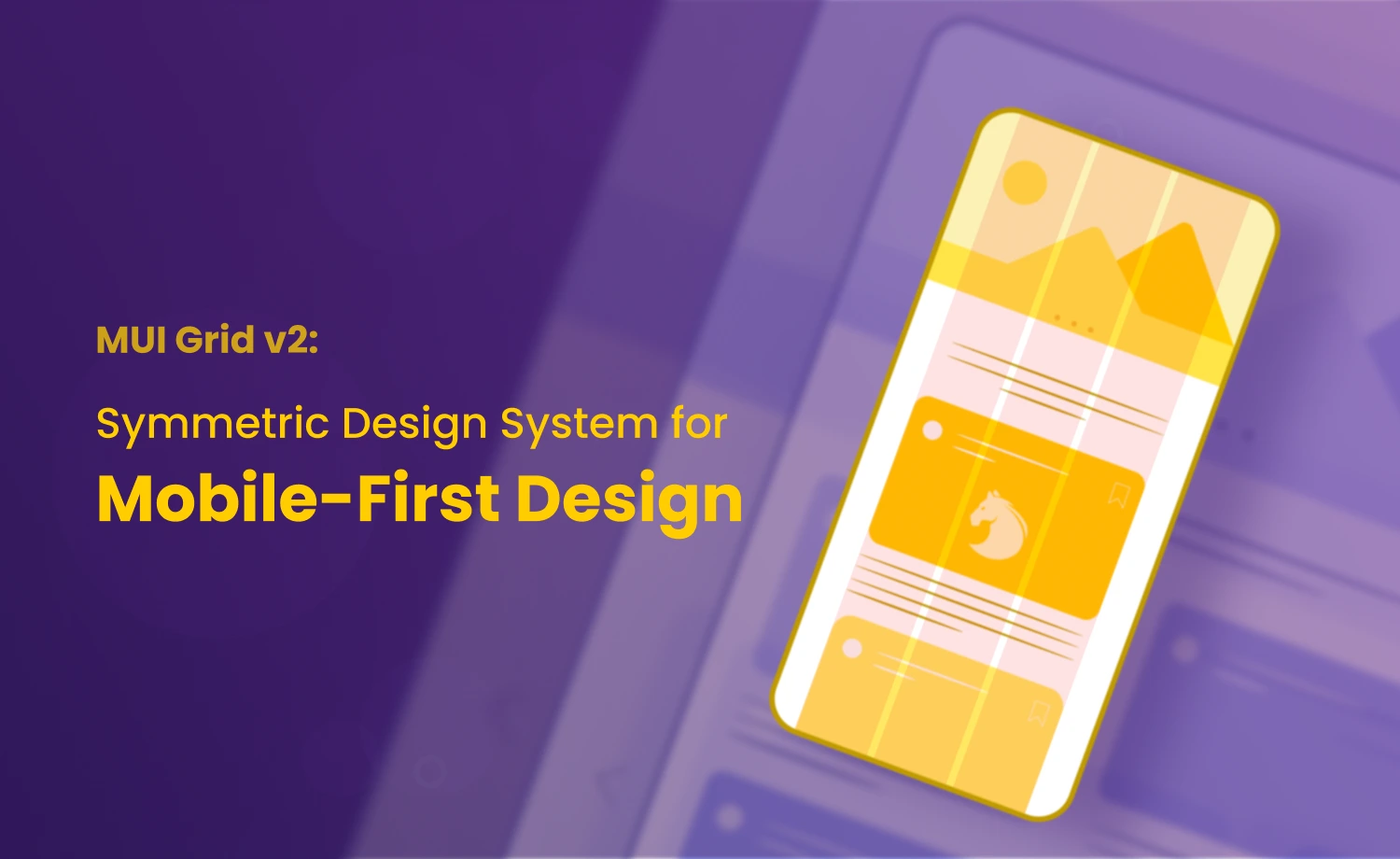
In web design, a grid system is the ideal way to establish any web application’s aesthetic value and user experience. To meet the accelerating demand for mobile-first web designs among businesses and stakeholders, popular web design frameworks, including Material UI (MUI) are working to develop responsive design systems.
This article will emphasize the latest version of MUI grid- Material UI Grid v2. The new MUI Grid is more developer-friendly and offers smoother customization options. Additionally, we will cover everything from ways to migrate from the older versions to the latest, to the basic customization concepts of the MUI grid system, exemplifying with a simple responsive card design with it.
In this blog, we will uncover the Material UI Grid System to help you create visually consistent layouts without blemishing the flexibility of the web pages. It will further help you enhance and optimize the performance of your websites.
Table of Content
What is Material UI Grid System?
The large component library of Material UI avails a distinct Grid component to facilitate your MUI project. You can establish an MUI Grid System, incorporating the Grid component in your project.
Additionally, MUI Grid v2 uses the CSS Flexbox for better flexibility. So, a basic understanding of the way CSS Flexbox works may provide additional insight into the MUI grid system.
Previously, the MUI Grid system had two properties: containers and items. The grid container was the parent or wrapper that organized child components (grid items) in a flex box-like layout. It used to contain one or more grid items with spacing, alignment, styling, or other properties. Grid items referred to the child elements that availed a certain amount of grid layout and specified a particular element’s behavior on the viewport.
To make coding more efficient, the updated version MUI Grid v2 has introduced a grid that functions simultaneously as an item and a container. We will discuss it elaborately in the following sections.
:: Remember that, the MUI Data Grid and the Material UI Grid component are two completely different things. MUI Data Grid displays tabular data from a dataset, while MUI Grid component is actually a layout grid for creating responsive layouts.
Starting with the Installation of MUI Grid v2
Before using the MUI Grid v2, you need to add the “@mui/material” package to your project.
npm install @mui/material @emotion/react @emotion/styled
// or,
yarn add @mui/material @emotion/react @emotion/styled
After adding the package, import the grid component using the following:
import Grid2 from '@mui/material/Grid2';
// or
import { Grid2 } from '@mui/material';
Material UI Grid v2 Container prop for Responsive Design
As mentioned above, Grid is now an item in MUI Grid v2. The grid will act both as a container and an item, like a flex item, always in the latest MUI Grid. Grid items refer to the child elements that take a certain amount of grid layout and specify a particular element’s behavior on the viewport. Instead of the item prop, you can use the container prop and implement the necessary customization.
Follow the example below:
import React from 'react';
import { Grid2 } from '@mui/material';
function MyComponent() {
return (
<Grid container>
<Grid {sizing or spacing values here}>
{Content goes here}
</Grid>
</Grid>
);
}
Migrating to Material UI Grid v2
The older version of MUI Grid is deprecated, and it’s recommended that you migrate to Grid v2 for smoother use with intuitive layouts for your project. If you’re curious about why you should use the updated version and migrate, check the following paragraph. Or else, you might want to to skip it.
Why You Should Migrate to Material UI Grid v2
The latest Grid v2 offers several changes, ease of customization, and fixes difficulties of previous versions. The key considerations are listed below:
- In the MUI Grid v1, there were some limitations with negative margins, spacing issues with full-width items or layout shifting, not creating proper gutter in v5, etc. These problems are fixed in Material UI Grid v2.
- The Grid component has been completely rewritten with calc() and CSS variables in Grid v2. As a result, the negative margin spreads equally on all sides by default.
- In MUI Grid v2, Grid is an item itself, which works similarly to the Flexbox in CSS. This new Grid2 automatically handles the size of long texts for its container. So, the item and zeroMinWidth props are removed, and you can define breakpoints, spacing, or customization values for your layout with less code.
-<Grid item zeroMinWidth xs={6}>
+<Grid xs={6}> - The latest version also fixed the limitation on nested grids and added the offset prop, which refers to adding space or gaps before a grid item. This ensures the item is positioned further along the row without content directly adjacent to it.
- A new prop called
disableEqualOverflow
is introduced, which solves the issue of unwanted scrollbars appearing on small viewports. It also lets you use the negative margins as the Grid v1 if needed.
Now that you know about the latest version of MUI Grid, it’s time to start the migration part. Remember that, there are different MUI versions available, and the migration steps and APIs vary for each.
Migration for Material UI v4 and v5
The MUI Grid v2 was introduced in MUI v5. So, if you are a Material UI v4 user, you need to migrate Material UI v4 to Material UI v5 to use the MUI Grid v2. Let’s see how to do that:
- First, upgrade the React.js and Typescript versions. Your React version should be updated to at least v17.0.0; if you’re using TypeScript, update it to at least v3.5. Also, update the packages below if your project is using them: “react-scripts,” “@types/react,” and “@types/react-dom”.
npm install @material-ui/core@^4.11.2 react@^17.0.0
or,yarn upgrade @material-ui/core@^4.11.2 react@^17.0.0
:: Use at least the Material UI v4.11.2 package and make sure all applications are running correctly before starting all the steps. - Second, set up the ThemeProvider at the root of your app.
- Third, install the packages of MUI v5 using the following:
npm install @mui/material @mui/styles
or,yarn add @mui/material @mui/styles
- Install the new packages of @material-ui/lab or @material-ui/icons, if you’re using them.
For @material-ui/lab:npm install @mui/lab
or,yarn add @mui/lab
For @material-ui/icons:npm install @mui/icons-material
or,yarn add @mui/icons-material
- Add Emotion packages for the new peer dependencies in MUI v5.
npm install @emotion/react @emotion/styled
or,yarn add @emotion/react @emotion/style
Once the installation is done and still your app is running without bugs or errors, run <npm uninstall @material-ui/*> or <yarn @material-ui/*> to remove the old @material-ui/* packages.
When you are done migrating, you can import the following to get the updated Grid v2 –
import Grid from '@mui/material/Unstable_Grid2';
Migration for Material UI v6
If you are not a Material UI v6 user, it is recommended to migrate to Material UI v6 from Material UI v5 first. It doesn’t take much time, and it is more fun to work with Material UI v6. When you’re a Material UI v6 user, you can follow the guidelines below:
The Grid component has been stabilized and is marked as stable in Material UI v6, so the unstable prefix for the Grid is removed in MUI v6. You can use the following:
import Grid from '@mui/material/Grid2';
//or
import { Grid2 } from '@mui/material';
Besides the stabilization, MUI v6 has also been improved with Grid v2 properties. The size
and offset
properties for corresponding breakpoints have been renamed. Hence, writing code has become easier with MUI v6, enhancing the development experience.
<Grid size={{ xs: 12, sm: 6 }} offset={{ xs: 2, sm: 3 }}>
You can also use custom breakpoints using the following
<Grid size={{ mobile: 12, desktop: 6 }} offset={{ mobile: 2, desktop: 4 }}>
It will ensure the viewports for mobile and desktop screens are occupied according to given breakpoint values.
Moreover, v6 removes the need for the disableEqualOverflow
prop for the Grid component, as the child Grid, correctly contains its parents’ padding.
If you need more details about migration to MUI Grid v2, please check the migration guide.
Limitation
Despite fixing some major issues of the previous version of MUI Grid, the size and offset props are not supported within the containers using direction="column”
and direction="column-reverse.”
So, the column and column-reversing issues remain.
The size
and offset
props define the number of columns a component uses for a given breakpoint and control the width using flex-basis in row containers. However, they impact the height of the column containers and may unexpectedly affect the height of the grid item elements.
Incorporate Material UI Breakpoints in Grid v2 for Intuitive Layout
MUI grids are an excellent tool for precisely and uniformly spacing items on web pages. Each grid width is a specific percentage of the grid’s 12-column layout. Additionally, MUI has breakpoints used internally in the components to create responsive layouts. They ensure the column widths adapt to various devices without breaking the orientation. You can control the layouts of your application through the Grid component. The default breakpoints for different screens and the available props for utilizing those are as follows:
Breakpoints
xs
, for extra-small screens: 0-599 pxsm
, for small screens: 600-899 pxmd
, for medium screens: 900 pxlg
, for large screens: 1200 pxxl
, for extra-large screens: 1536 px
Props
- size
- columns
- columnSpacing
- direction
- rowSpacing
- spacing
- offset
Look into the example of Grid customization with the breakpoints.
import React from 'react';
import { Grid2 } from '@mui/material';
function MyComponent() {
return (
<Grid container size={{ xs: 4, sm: 8, md: 12 }}>
{/* your content here*/}
</Grid>
);
}
In this example, we created a simple grid container for extra-small, small, and medium screens that take width according to the values given. The grid container occupies approximately 34% {xs:4}
of the smaller or extra-small screen’s viewport, where it occupies approximately 67% {sm:8}
for a small screen and 100% {md:12}
for a medium screen’s available viewport.
Details on the Features of MUI Grid v2
MUI Grid v2 includes the same features as MUI Grid, such as the fluid grid, spacing, auto-layout, and nested grid. Let’s get a grasp on the MUI Grid v2 features
Fluid Grid
The fluid grid in Material UI is a layout that dynamically scales the contents of your webpage. It utilizes the breakpoints to determine the requirements for the layout modifications. A fluid grid expands the layouts based on available spaces by adjusting proportionally to different screen widths. For example, <size={{ xs: 12, sm: 6 }}>
occupies 100% of the available viewport of the small screens (xs
) like mobile, as it is defined xs:12
, (12 out of 12 columns = full width), and for the screens like tabs (sm
), which is bigger than a mobile (xs
), the component occupies half of the total viewport.
Check the example below, where we’ve created two grid items that change the layout at the defined breakpoints:
Spacing
Spacing controls the space or gutter between the grid items. It adds padding inside the container and ensures the items don’t stick together. You can utilize this feature using the spacing
prop. And if you want to convert it into CSS property, use the theme.spacing()
helper.
See the following example for a better understanding:
Here, the vertical space between the grid items will be 8px (as the spacing is 8px by default) and the horizontal space will be 8px for the extra-small screens, 16px (2*8) for the small screens, and 24px (3*8) for the medium screens.
Auto-Layout
The grid items can automatically resize and fill the available space using the auto-layout feature. It eliminates the need to specify an item’s width. If the width of one of the items is set to a fixed value, the remaining child items will automatically resize and share the available space for them.
For instance:
In this example, there are three child items, and the size for the second one is defined. The remaining two children will automatically occupy the available space to align with the viewport.
Nested Grid
The nested grid feature of the Material UI Grid System is generally used for complex structures or designs. A nested grid container must be the direct child of a root or parent grid container. Otherwise, if any items are defined between them, the second grid container will act as the new root container.
See the example below:
<Grid container>
<Grid container> // A nested grid container that inherits columns and spacing from above.
<div>
<Grid container> // A new root grid container with its own variable scope.
The nested grid container inherits the columns
and spacing
props. If these props are not specified for the instances, the child grid inherits the row spacing and columns from its parent.
Customizing Material UI Grid Column Number
MUI allows you to customize column numbers when implementing designs that do not use the default 12-column grid. You can use the columns prop to change the number of columns. It helps you get more flexibility.
See the following example to see how it’s done:
It will create 15 columns with items A and B, where A takes around 47% and B takes around 53% of the screen width.
Making a Simple Responsive Card with Material UI Grid v2
Since we have learned quite a lot about MUI grid systems and grid v2, let’s explore making a real-life example like a responsive card.
This example creates an image gallery with 6 cards and provides a responsive layout for different viewports.
As Johann Wolfgang von Goethe said, “In the end, we retain from our studies only that which we practically apply.” This blog has enlightened you on the basic usability of the powerful grid system of your favorite Material UI and how to utilize it in your project without getting confused about the core concepts.
So, it’s time to put your knowledge to testing and deployment and transform your developer experience from beginner to master. Start with the prebuilt Material UI dashboard templates and enjoy hassle-free development process along with complete customizability.
Material UI Grid v2: FAQs Answered
The Material UI Grid uses CSS flexbox rather than the CSS grid. But the latest versions of MUI grid don’t support row spanning. So, to span the children elements in multiple rows, MUI grid uses a CSS grid. The MUI grid also uses CSS grid when needing an auto-placement of children element.
Yes, the MUI Grid is a React component provided by the Material-UI (MUI) library. It is part of the MUI system for building responsive layouts using CSS flexbox under the hood. Grid is a system to build responsive layouts, and it is implemented using the grid component.
Yes, in the case of nested grids, the MUI grid can act as the container and item. The outer grid acts as a container, the inner grid acts as both an item (inside the outer container) and a container (for nested items).
Explore more to expand your knowledge on Material UI