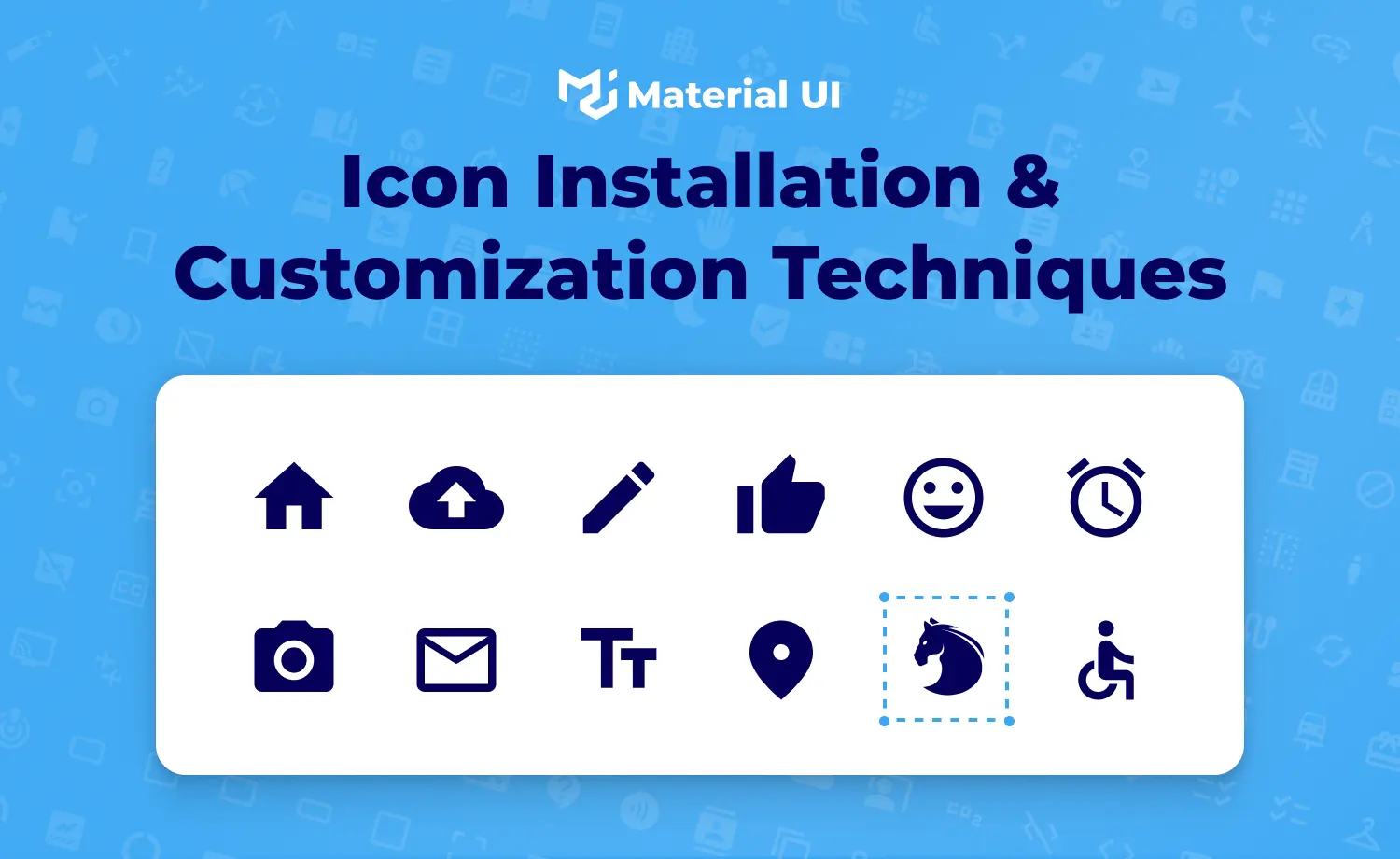
Material UI has a massive UI component library, inspired by Google’s Material Design system, to empower React developers. These multi-type components have built-in designs, which assist developers in creating extraordinary designs. MUI icon is one of them. These icons help the design represent the tone of the author’s or company’s in a more effective way.
This blog post will discuss how to make the most of the MUI icons in your project, discussing step-by-step integration styling.
Table of Contents
Getting Started with MUI Icons
Material UI library contains 2100+ material icons converted into SvgIcon components available under the “@mui/icons-material” package. These icons use the Material UI SvgIcon component to render the SVG path for each icon. Let’s begin installing the MUI icons.
Material UI Library Installation
Installing Material UI library with a React-based project is generally easier. To utilize Material UI icons in your project, you need to install the Material UI library as the “@mui/icons-material” depends on the “@mui/material” package. However, before installing the Material UI library, you must install the following dependencies:
"peerDependencies": {
"react": "^17.0.0 || ^18.0.0",
"react-dom": "^17.0.0 || ^18.0.0"
},
When you have these peerDependencies
installed, it’s time to download and install the MUI icon packages. It is very useful, especially when importing several icons simultaneously. For installation of this package in your directory, use the code below:
// with npm
npm install @mui/icons-material
// with yarn
yarn add @mui/icons-material
Installing MUI SVG Icon API
If you want to use MUI SVG icons, you can use the SvgIcon
wrapper. This package provides the Google Material Icons converted to SvgIcon
components. It comes with built-in accessibility.
For installation use:
import SvgIcon from '@mui/material/SvgIcon';
// or
import { SvgIcon } from '@mui/material';
Besides, MUI allows you to use custom SVG icons using the MUI SvgIcon
wrapper. It will be covered in the following sections.
Importing Material UI Icons
After installing the “@mui/icons-material” package, you have full access to the entire Material UI icon set. To import the icons in your project, add an import
statement at the top of your project file, following these 2 options:
Option 1
import AccessAlarmIcon from '@mui/icons-material/AccessAlarm';
import ThreeDRotation from '@mui/icons-material/ThreeDRotation';
Option 2
import { AccessAlarm, ThreeDRotation } from '@mui/icons-material';
If you want to import the icons by reducing bundle size, option 1 is the safest way. But some developers also choose option 2. After that, simply use the icon as a component in your JSX code, like this: <AccessAlarmIcon />
.
For example:
Each Material UI icon includes 5 built-in themes. They are: filled (default), Outlined, Rounded, Two-tone, and Sharp. While importing these components, you can add these theme names to the icon name and get a default shape for the icons. For example, @mui/icons-material/MenuRounded
gives a hamburger icon with a rounded theme.
See the following examples:
Customizing Material UI Icons
Like the most popular frameworks, Material UI icons have a built-in design and don’t require much modification. Yet, it’s better to change the color and size slightly to help it fit well with your design. Now, we will look at customizing the color and size of the MUI icons.
How to Change Material UI Icon Color
MUI icons by default inherit the original text color. However, you can customize icon color using the theme color properties (primary, secondary, action, error, and disabled) or using color codes.
Here, we get 5 home icons where the 1st icon color is the default original text color, the 2nd to 4th icon has the color according to the color properties, and the last one is pink as it was customized with color code.
How to Change Material UI Icon Size
The default size of the MUI icons is 24px. But you can modify it using the style property to add custom CSS font-size values such as “small,” “medium,” “large,” “inherit,” and “default.” You can also set a fixed size by using a specific number value.
From this code example, we get 3 home icons with different sizes. Since there was no customized color set, it took the original text color, and the 1st one has the default size. The other 2 icons are large, and customized fixed value size: 50 accordingly.
Additionally, you can also adjust the size using the fontSize
property of the IconButton
component. It allows specifying the size of the icon within the button itself.
How to use SVG Icons in Material UI
Material UI lets you create customized icons according to your branding style. The MUI icon set has createSVGIcon
utility component that allows you to create custom MUI SVG icons for your project. Take a look at the following example:
Here, the createSvgIcon
utility component wraps the <svg> element and the SVG path. The MUI SvgIcon
component then inherits it as a child.
Using Material UI Icon
Component
React allows the Icon
component to add icons within Material UI. It displays any icon font that supports ligatures. The Icon
also inherits the current text color, but you can also set it differently, like other MUI icons. To use the Icon
component, simply wrap the icon name (font ligature) with it.
import Icon from '@mui/material/Icon';
<Icon>star</Icon>;
NB: When using the Icon font, it is important to include the Material Icons font as a prerequisite in your project.
This is an example of a font Icon.
How to Use Font Material Icons
The Icon component sets the correct base class name for Material Icons with the ‘filled’ variant. You just need to load the font you want to use, for example, Google Web Fonts. You can load the font using this:
<link
rel="stylesheet
href="https://fonts.googleapis.com/icon?family=Material+Icons
/>
To use other fonts, you can use custom fonts. For this, customize the baseline class using the baseClassName
prop.
For example, if you want to use two-tone icons using the Icon component, load the font like this:
import Icon from '@mui/material/Icon';
<link
rel="stylesheet
href="https://fonts.googleapis.com/css?family=Material+Icons+Two+Tone
/>
It will import the two-tone Material Design variant.
You can also declare the baseClassName
prop globally. It will let you use fonts directly and prevent you from constantly changing the prop, which will help you perform better.
const theme = createTheme({
components: {
MuiIcon: {
defaultProps: {
// Replace the `material-icons` default value.
baseClassName: 'material-icons-two-tone',
},
},
},
});
<Icon>add_circle</Icon>
This code snippet displays two-tone icons with Material Design and lets you design the font directly.
How to get the best out of a MUI Icon Set
Customizing MUI icons is necessary to match your branding or design approach. But there arises some misuse of the MUI icon set too. So we should follow some tips to get the best out of it:
- Understanding Requirements: Gather your design requirements and goals. It will help you proceed with the suitable icons you need.
- Importing Icons Individually: Importing icons individually helps optimize bundle size. So import only the icons you need. Example:
import HomeIcon from '@mui/icons-material/Home';
import SearchIcon from '@mui/icons-material/Search'
- Using Correct Version: MUI icons also have different versions to work seamlessly with the corresponding MUI version. So, you must ensure your MUI icon set’s version matches your core version.
- Accessibility: When necessary, use the {aria-label} or {title} attributes to make icons easier to read and use with screen readers.
- Follow Design Consistency: Maintain consistency in using the icons throughout the application to ensure a cohesive design.
In conclusion, we’ve gone from understanding the fundamentals of Material UI icons to delving deep into the world of SVG icons to how these small design elements can change things. Although the world of icons may appear big and challenging to understand, it’s a fun place with lots of options if you know what you’re doing and have the right tools. Material UI provides versatile customization with its components and tools, so it becomes fun with MUI icons as well.
If you’re trying to explore Material UI for your next project, a better approach could be to find pre-built templates for a simpler access into the framework. Moreover, for a better insight into the framework and to find answers to the frequently asked questions about Material UI, explore our article on Material UI FAQs.