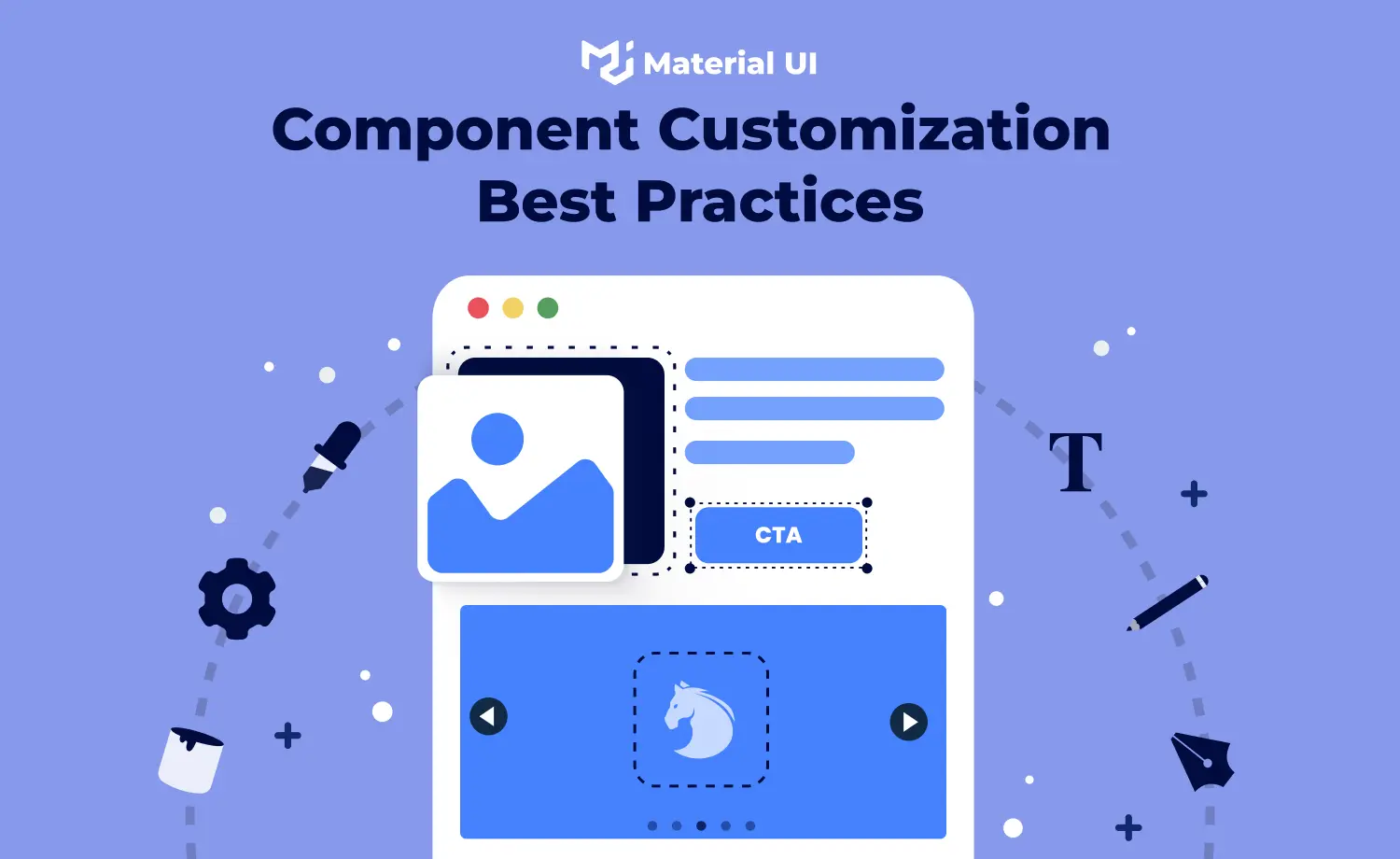
Material UI (MUI) is a massive UI component library that offers multiple customization styles for building polished, professionally rooted websites. But sometimes the MUI component customization becomes overwhelming with the steeper learning curve.
In my opinion, learning about the component customization practices of a UI library is one of the easiest ways to learn about the component library quickly. That is why we’re going to talk about MUI component customization today. We’ve gathered some simple ways of Material UI component customization with examples so you don’t get confused between the styles and your learning becomes smooth. Happy Learning!
Table of Content
How to Customize Material UI Components
MUI components have props that allow fine-tuning their appearance and behavior for consistent design. Generally, these props have default values, so it doesn’t require much customization for the components.
But what if you could use a different font size for the typography or change the shadow of a card component? Or you could create your own customized theme for your application?
Material UI components contain these flexibilities that you can customize and re-shape them in however way you want. But, it really won’t be a good practice to play too much with these customization advantages. If you want to try varieties tailoring with MUI, then it mayn’t work as expected. Go through our other article on choosing the right framework that suits your project goal.
The component customization styles of MUI include many approaches like using theming options, creating theme object, style overrides, the sx prop, CSS theme variables, and styled() API. Let’s get started understanding these approaches.
Theming & Styling with MUI Theme Object
Styling is a critical aspect of UI development. On the other hand, theming is a powerful tool in MUI and promotes greater consistency between apps. Each of these two approaches conveys higher performance and optimization.
Simplify Your Customization with Theming
MUI’s theming is one of its strengths for customization. It lets you customize or update the components globally. A theme in Material UI is an object describing the styling properties such as color, font sizes, shadows, opacity, and more. In theming, you can style by creating a theme object using createTheme
and applying it to the app using ThemeProvider
,( a component used to inject customized theme objects into your application). Moreover, you can customize all the MUI components in this way.
:: Note that ThemeProvider
must be a parent of the components you are trying to customize to pass down the customized theme to the components.
Style Overrides for Global Customization
The styleOverrides
key lets you change the default styles of a Material UI component. It requires a slot name like – root as a key and an object with CSS properties as a value. It is used to change the default styles of MUI component props and for global customization.
Overriding Styles Using TypeScript
Using TypeScript with Material UI styling can enhance your development experience by providing autocompletion, type checking, and other benefits. It ensures that you’re using your theme correctly and helps prevent bugs and errors in your code.
If you are using TypeScript, you need to specify your new variants or colors using module augmentation.
declare module '@mui/material/Button' {
interface ButtonPropsVariantOverrides {
dashed: true;
}
}
Variants Key
The variants
key allows for overriding styles in a particular slot for specific props. You may not find variants
in all MUI components, but you can add it to your components anytime. You can also create custom variant for overriding style. Here’s how you can do that:
In this code, we added a new variant “dashed” to the button component, which is not available in the Button API. The new variant is specified using the module augmentation at first, then added to the button component. Next, the button with the new variant from the component path is called in the app, creating a theme object wrapped by ThemeProvider
.
Then, you can modify the existing variants
values in the MUI components, like overriding styles based on existing props or adding styles based on new values. Just make sure that the styles with precedence are listed last.
The example above demonstrates overriding styles for an existing variant props
(color: secondary) and a new variant (variant: dashed) using variants
key.
However, it is best practice to avoid unnecessary overrides and use the provided props without compromising their default styles.
Callback in Styleoverrides
MUI provides callback support in overriding styles, which also facilitates global theming. It gives you full control of the component customization at the theme level.
In the latest MUI versions, the variant props
is defined as a callback. It allows you to style based on conditions and helps style when a property doesn’t contain specific values.
This is an example of callback using variants
key, where if the variant props is dashed and color is secondary, the button has the customized border and color.
Below is another example of callback support in MUI using MuiCSSBaseline
component with the styleoverrides
key.
See! Theming and styling is fun and flexible in MUI. You just need the component’s slot name and an object or a callback, and you’ve got it!
Styled() Function
The styled() function of MUI allows for custom-styled components and the reuse of the overrides in different parts of your application. It is part of the “@mui/system” packages and leverages the CSS-in-JS approach to styling in React.
import { styled } from '@mui/system';
// If you are using @mui/material
import { styled } from '@mui/material/styles';
This styling approach enriches MUI’s theming and creates maintainable, theme-aware, and modular components.
In this example, we’ve created a button with a hover effect. It overrides the default styling of the button component and take a different color, font size, and shape. You can remove the default shadow effect using disableElevation
prop.
In addition, the styled() function allows creating custom styled() utilities using the createStyled
utility.
import { createStyled, createTheme } from '@mui/system';
const Theme = createTheme({
// your custom theme values
});
const styled = createStyled({ Theme });
export default styled;
Furthermore, this styled()
utility emphasizes using reusable components to reuse the same overrides in different locations and dynamic overrides based on a component’s props. Thus, it enhances the developer experience with consistent customization for different apps and websites.
:: Note that, while using TypeScript
, update the prop’s types for the new component.
Add Dynamic Styles with Dynamic Overrides
The styled() utility facilitates adding dynamic styles to your components using dynamic overrides. You can use dynamic CSS or CSS variables for this.
Styling with Dynamic CSS:
import * as React from 'react';
import { styled } from '@mui/material/styles';
import Slider, { SliderProps } from '@mui/material/Slider';
interface StyledSliderProps extends SliderProps {
success?: boolean;
}
const StyledSlider = styled(Slider, {
shouldForwardProp: (prop) => prop !== 'success',
})<StyledSliderProps>(({ success, theme }) => ({
...(success &&
{
//the overrides are added when the new prop is used
}),
}));
Styling with CSS Variables:
<React.Fragment>
<FormControlLabel
control={
<Switch
checked={vars === successVars}
onChange={handleChange}
color="primary"
value="dynamic-class-name"
/>
}
label="Success"
/>
<CustomSlider style={vars} defaultValue={30} sx={{ mt: 1 }} />
</React.Fragment>
Using CSS Theme Variable in MUI Customization
CSS variables help improve MUI’s theming and customization, letting you declare variables in CSS and reuse them in other components.
To use CSS theme variables in your project, create a theme with cssVariables:true
and wrap your app with ThemeProvider
.
import { ThemeProvider, createTheme } from '@mui/material/styles';
const theme = createTheme({ cssVariables: true });
function App() {
return <ThemeProvider theme={theme}>{
/* ...your app */
}
</ThemeProvider>;
}
Take a look at the following example:
:: All features of the experimental CssVarsProvider
are available in the latest MUI versions (v6 to above) with ThemeProvider
, so you should use ThemeProvider
instead of CssVarsProvider
.
CSS theme variables in MUI leverage native CSS for styling efficiency. You can use it directly in CSS, JavaScript and styled components. To utilize CSS variables in themes, theme.vars
object is recommended instead of vars
. Let’s create a button using these CSS theme variables in styled utility.
:: Remember to avoid using vars as a property name when adding custom variables to your theme.
If you are using a pure CSS file and cannot access theme object, you can use var
directly like this:
Inline Styling with the sx
prop
The sx prop has been a stable feature for customizing components. It acts like the style tag in CSS and helps in the inline styling of MUI components. You can build theme-aware styling and improved responsiveness with sx without code difficulties. Let’s see an example for a button:
sx
Prop allows you to write codes without overriding styles. If you don’t find it suitable for your code and need to separate the styles, use the styled()
function for your expected outcome.
The sx
prop inside the styleOverrides
key modifies styles using shorthand CSS notation within the theme. This allows you to use the same syntax in your theme and quickly transfer between the two.
The sx
prop offers many theme-aware properties like border
, borderColor
, borderRadius
for borders of a design, displayPrint
to specify the CSS display
value applied only for printing, and CSS grid properties like gap
, rowGap
, and coloumnGap
, etc. You can get more about the sx
prop in this doc.
Additionally, the sx
prop is a stable customization feature, but it is considered experimental when used inside the themes directly. However, its higher CSS specificity allows you to override the components even with the experimental sx
syntax when you customize them using the theming approach.
Limitations of MUI Component Customization
Although MUI customization is powerful, there are a few reasons why it can occasionally be difficult. Some key factors are:
- One of the primary challenges is understanding the extensive theming system and the various ways to apply styles, such as using the sx prop, styled utility, or custom themes, which can be overwhelming for beginners.
- Sometimes the default design language of MUI may not align with the design requirements. Sometimes combining global styles, component overrides, and custom styles can also be tricky. All these things result in integration difficulties of custom design while keeping component consistency.
- Performance consideration is another one that can arise when applying complex styles or using custom components, making it essential to balance customization with efficient rendering.
Now, you may be wondering how much customization MUI can afford. The answer was mentioned earlier. It is ok to customize a little the components to fit with your design goals, but if you need to customize a lot, then maybe MUI is not the right framework for you, especially when you don’t need material design. Additionally, if you want to customize a little bit more, that should be done using inline or custom styles, not defining multiple themes.
We are almost done!
MUI customization can significantly elevate your web application’s user interface, giving it a unique look that aligns with your brand identity. It is designed to be automatically responsive, adjusting to different screen sizes, which also helps optimize the layout’s performance. Whether you want to tweak a few colors, adjust typography, or completely maintain the component styles, MUI provides a versatile and powerful customization system that serves all levels of developers.
FAQs : MUI Component Customization
MuiButton in a theme enforces consistent styles for all Button components across your application. And the MUI Button component is for direct usage in your component tree. It is used to display buttons in your app.
The sx prop in MUI works for inline styling or one-off customization in MUI. You can use it in all MUI components. But it is not recommended to use for complex coding. The sx prop is suitable for minor customizations but it may create inconsistencies in the app’s look and performance
makeStyles
and withStyles
in MUI v5? The makeStyles
and withStyles
hooks were part of the “@mui/styles” package, maintained alongside MUI v4. However, the updated versions (v5 and above) do not recommend using these hooks.